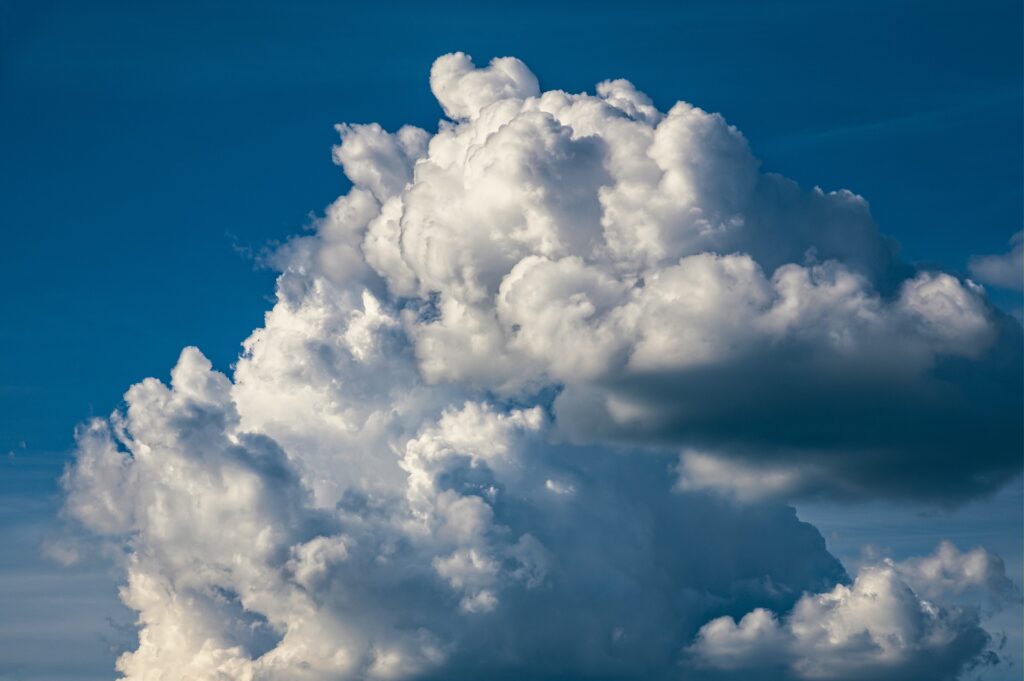
What is Hoisting in JavaScript?
Javascript Hoisting refers to the process of moving functions,variables and classes to top of their scope before execution. JavaScript interpreter is responsible for this hoisting. By doing this, we can use variables and functions in code before they are declared. Hoisting should be used carefully as it can lead to unwelcomed errors if used in certain conditions.
Let’s see in detail each type of hoisting in javascript.
Variable Hoisting
As you know, variables can be accessed before their declaration as the result of hoisting process but, only declaration is hoisted, not the initialization. Confusing right? Let’s see it with an example.
console.log(article); // value will be undefined
var article = 1;
You can see, that we are accessing article variable before its initialization and declaration. This is because JavaScript moved declaration of article to the top so this code will not cause any error. You can see the value is undefined and not 1 because the default initialization of var is undefined.
Logically, the above code will look like the below after the hoisting
var article;
console.log(article); // value will be undefined
article = 1;
Hoisting with let and const variables
Variables declared with let and const are also hoisted but are not initialized with undefined default value. Therefore, below code will throw an error.
console.log(article); // Throws ReferenceError exception as the variable value is uninitialized
let article = 1; // Initialization
Above code will throw the below error:
ReferenceError: article is not defined
Note that if we forget the declaration (and only initialize the value) the variable isn’t hoisted.
Function hoisting
Function hoisting allows using the function before its declaration. Let’s see it with an example.
showName("CodeTopology");
function showName(name) {
console.log(`I Love ${name}`);
}
Note that, functions expressions and arrow functions are not hoisted.
Class hoisting
Classes declaration are hoisted in JavaScript, which means that JavaScript has a reference to the class. However the class is not initialized by default, so if you try to use it before initialization will throw a reference error. Similar to function expressions, class expressions are not hoisted.