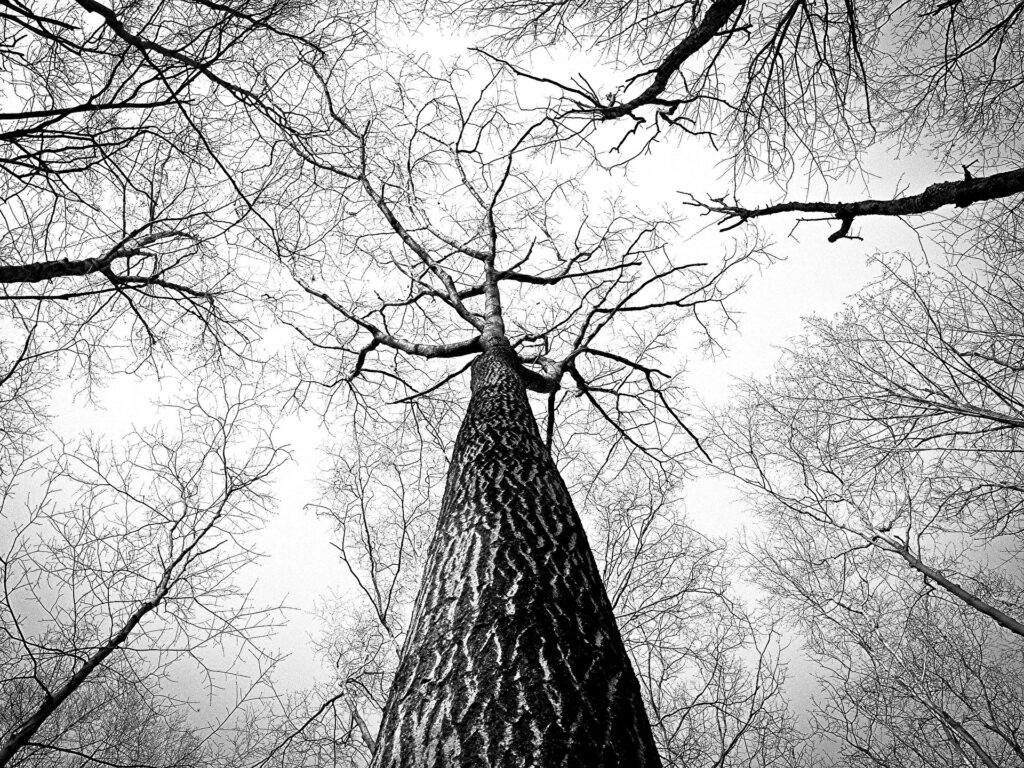
What is DOM in JS?
DOM stands for Document Object Model and is a programming interface that is used to access, edit, delete, and create elements in JavaScript. It represents the logical structure of the HTML or XML document. You can bind events to HTML elements using DOM. DOM treats HTML documents as a tree and views each element as a Node.
Let’s see with an example how DOM interprets HTML documents:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Code Topology DOM tree structure</title>
</head>
<body>
<h1 id="titleHolder">DOM tree structure</h1>
<h2 id="secondTitleHolder">JavaScript Tutorials</h2>
<p class="paraHolder">Line 1</p>
<p class="paraHolder">Line 2</p>
</body>
</html>
In the above example, the document is treated as a root node, HTML is a child node, HEAD and BODY are children of HTML nodes. Let’s see it in the diagram:
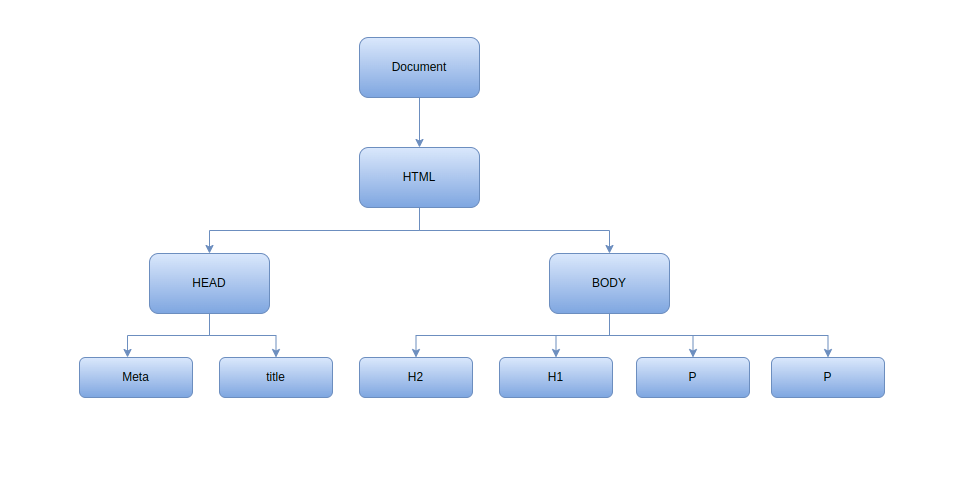
As you can see, the BODY tag has H1, H2 and P as a child and HEAD tag has meta and title tags as a child.
As you know, we can access the elements in DOM with Javascript, Let’s see how to achieve that.
There are multiple ways in which we can access DOM elements, but we will focus on 2 methods in this article.
- getElementById()
- getElementsByClassName()
getElementById() takes a single argument, and that is the unique id of the element. If 2 elements have the same id, then this method will return the first element.
It will return null if the element is not present, or returns the element if an element is present.
const heading1 = document.getElementById("titleHolder");
console.log(heading1);
The console output of above line would be,
<h1 id="titleHolder">DOM tree structure</h1>
Now, Lets see second method – getElementsByClassName().
getElementsByClassName() takes one single argument – class names. If you want to access elements with different class names, provide it with a whitespace. This method will return HTMLCollection, which means, it returns the array of the elements. The elements in a collection can be accessed by index (starts at 0).
const collection = document.getElementsByClassName("paraHolder");
for (let i = 0; i < collection.length; i++) {
console.log(collection[i]);
}
The output of above code would be,
<p class="paraHolder">Line 1</p>
<p class="paraHolder">Line 2</p>
How to create dynamic element in JavaScript?
You can add dynamic element to DOM in JavaScript using document.createElement() method. This method takes the one argument and that is the type of element which we want to add.
const para = document.createElement("p");
para.innerText = "This is a dynamic paragraph added";
document.body.appendChild(para);
Above code will add p tag with specified text to the body tag of the document. You can even append the dynamically created element to existing element.
document.getElementById("existingElementId").appendChild(para);
Note that, if you only use createElement() method on document object without appending it to body or existing element, it will not get added to the DOM.
How to attach event to DOM element using addEventListener() method
Let’s assume we have a button in DOM and we need to show alert if user clicks on the button.
<button id="btnDemo">Click Me!</button>
We will use addEventListener() method to bind the click event to this button element.
const button = document.getElementById("btnDemo");
button.addEventListener("click", () => {
alert("Clicked this Button");
});
This method takes 3 arguments – first one is event name and second one is function which will get executed as the result of the event. Third argument is the useCapture of boolean type which is optional argument.