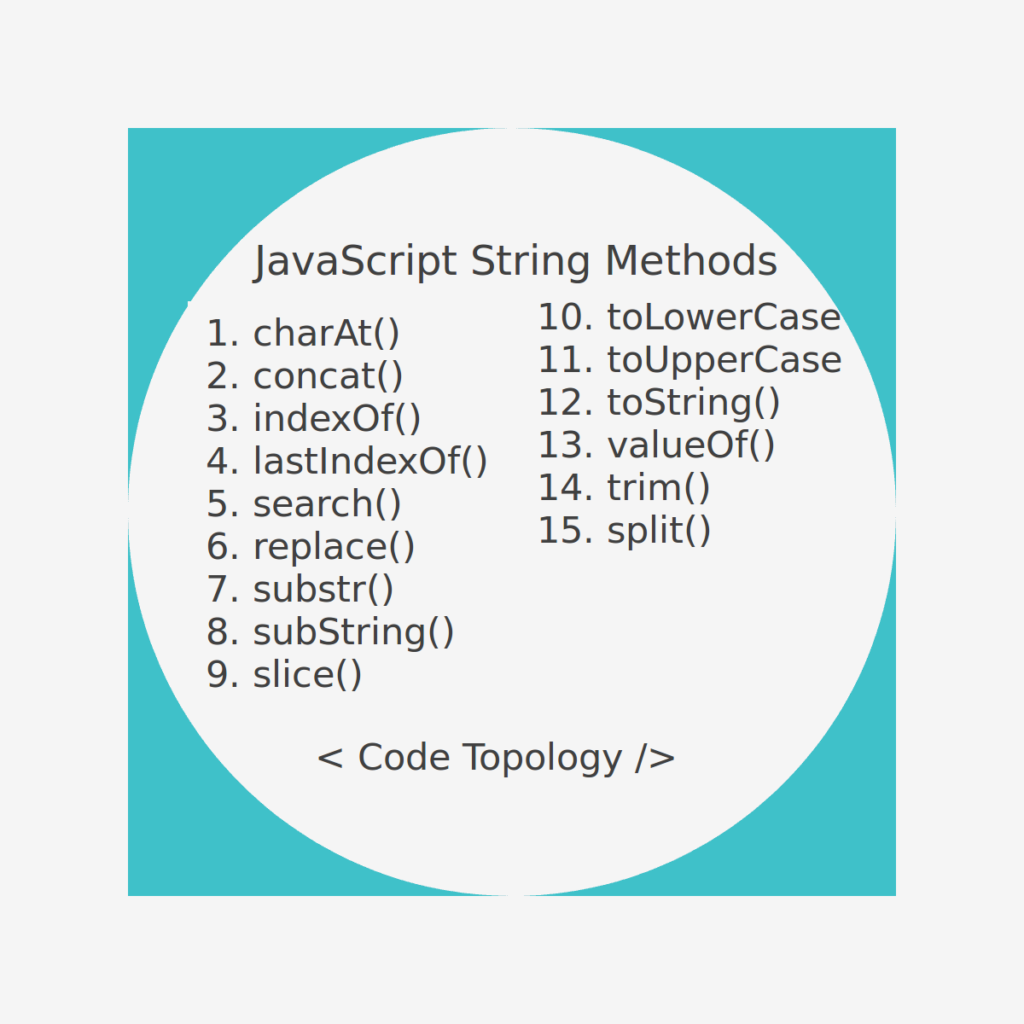
JavaScript string methods allows us to perform operations on string literals and String Objects. There are more than 20 string methods available in JavaScript. Out of those methods, 15 are most useful in day to day programming activity.
charAt(index)
charAt() returns a character at the specified index in a string. If specified index is not within string length, It will simply return empty string character.
Example of chartAt(index) is
var str = "Code Topology";
str.charAt(5);
// It will return T
concat(str1,str2,…strN)
concat() in Javascript combines 2 or more string and returns a connected string. There is no max limit on the number of arguments this method can take. It does not change original string on which concat() is invoked.
Example of concat() is
var str1 = "John";
var str2 = "Doe";
var finalOutput = str1.concat(" ", str2);
console.log(finalOutput); // It will return John Doe
indexOf(string)
indexOf() returns a position of the first occurrence string on another string.
If string is not found, it returns -1.
Example of indexOf() is
var str = "first will be returned first";
var pos = str.indexOf("first");
console.log(pos);
//output will be 0
lastIndexOf(text)
lastIndexof() returns a last occurrence of specified text in string. If string is not found, it will return -1.
Example of lastIndexOf()
var str = "last index of this 'last'";
var pos = str.lastIndexOf("last");
console.log(post);
//output will be 20
search(regex)
search() method is used to search a string within a string and get its position. It can take a primitive string as an argument or we can pass a powerful regular expression as an argument. search() also returns a first occurrence of string same as indexOf(). If no string is present, it will return -1.
You can learn more on regex on Regexr, It’s a great tool to play with regex online.
Example of search()
//Example with string as an argument
var str = "search me with search()";
var pos = str.search("search");
console.log(pos);
//output will be 0
//Example with regex as an argument
var str = "abc123";
var pos = str.search(/[0-9]+$/);
console.log(pos);
//output will be 3
Difference between indexOf() and search()
You might be thinking indexOf() and search() are same but its NOT. Here are the difference between indexof() and search() 🙂
- indexOf() can not work on regular expressions, Yes, we can not pass regex as an argument to indexOf()
- We can not specify starting index from where to start search in search()
replace(originalString,newString)
replace() method replaces original value with new value within string. It can also take regex as a first argument.
Example of replace()
var str = "replace this with chair";
var result = str.replace("this", "table");
console.log(result);
//output will be replace table with chair
substr(start,length)
substr() is used to extract part of the string. It returns string starting from position start and will return the length of string after that. If second parameter is skipped, it will return complete rest of the string.
We can also pass negative index as a start argument, so it will start position count from the end.
Example of substr()
//Example with second argument
var str = "Extract important data";
var result = str.substr(8, 9);
console.log(result);
//output will be important
//Example without second argument
var str = "Extract important data";
var result = str.substr(8);
console.log(result);
//output will be important data
substring(start,end)
substring() will return string starting at position start and ending at position end. If no end parameter is specified, it will return complete string.
Example of substring()
var str = "return this substring";
var result = str.substring(12, 21);
console.log(result);
//output will be substring
Difference between substr() and substring()
- Second argument in substring() is an index where to stop, not including the character at that position.
- The second argument in substr() specifies length of string to be returned.
- substr() can accept negative index as an argument to read string from end.
- substring() does not support negative index as an argument.
slice(start,end)
slice() in javascript returns a string starting at position start and ending with end position. slice() accepts a negative index, therefore, the difference between slice() and substring() is that substring() can not accept negative index as an argument.
Example of slice()
//slice example
var str = "return this substring";
var result = str.slice(12, 21);
console.log(result);
//output will be substring
//slice example with negative index
var str = "return this substring";
var result = str.slice(-10, -6);
//output will be sub
toLowerCase()
toLowerCase() method in javascript converts a string to lower case format.
Example of toLowerCase()
var str1 = "Code Topology";
var str2 = str1.toLowerCase();
console.log(str2);
//output will be code topology
toUpperCase()
toUpperCase() converts a string to upper case format.
Example of toUpperCase()
var str1 = "Code Topology";
var str2 = str1.toUpperCase();
console.log(str2);
//output will be CODE TOPOLOGY
toString(base)
toString() in javascript is used to convert number, arrays to string. It takes one optional argument as a number system base (2,8,16).
Example of toString() to convert number and array to string
//convert number to binary number system.
var str =121;
var result = str.toString(2);
console.log(result);
//output will be 1111001
//convert array to string
var str = ["abc","pqr"];
var result = str.toString();
console.log(result);
//output will be abc,pqr
valueOf()
valueOf() method in javascript will return a value of a string on which it is called.
Example of valueOf()
var str = ["abc","abc"];
var result = str.valueOf();
console.log(result);
//output will be abc,abc
trim()
trim() method is used to remove white spaces from both the ends of a string. Please note that it will not remove spaces within string if any.
Example of trim()
var str = " I am in the middle ";
var result = str.trim();
console.log(result);
//output will be I am in the middle
split(splitOn)
split() method in javascript is used to convert a string to an array. It takes one optional argument, as a character, on which to split.
If splitOn is skipped, it will simply put string as it is on 0th position of an array.
If splitOn is just a “”, then it will convert array of single characters.
Example of split()
//split on ,
var str = "a,b,c";
var arr = str.split(",");
console.log(arr[0]);
//output will be a
//split on
var str = "abc";
var arr = str.split("");
console.log(arr[0]);
//output will be a
That’s it!!
We have covered most of the javascript string methods. Let’s cover useful array methods in javascript here.
So here are some popular FAQs on this topic
Difference between indexOf() and search()
indexOf() can not work on regular expressions, Yes, we can not pass regex as an argument to indexOf()
We can not specify starting index from where to start search in search()
Difference between substr() and substring()
Second argument in substring() is an index where to stop, not including the character at that position.
The second argument in substr() specifies length of string to be returned.
substr() can accept negative index as an argument to read string from end.
substring() does not support negative index as an argument.
Does substr(), substring(), split() changes original string?
No, these functions will returns final output but will not change original string on which they are triggered.
One thought