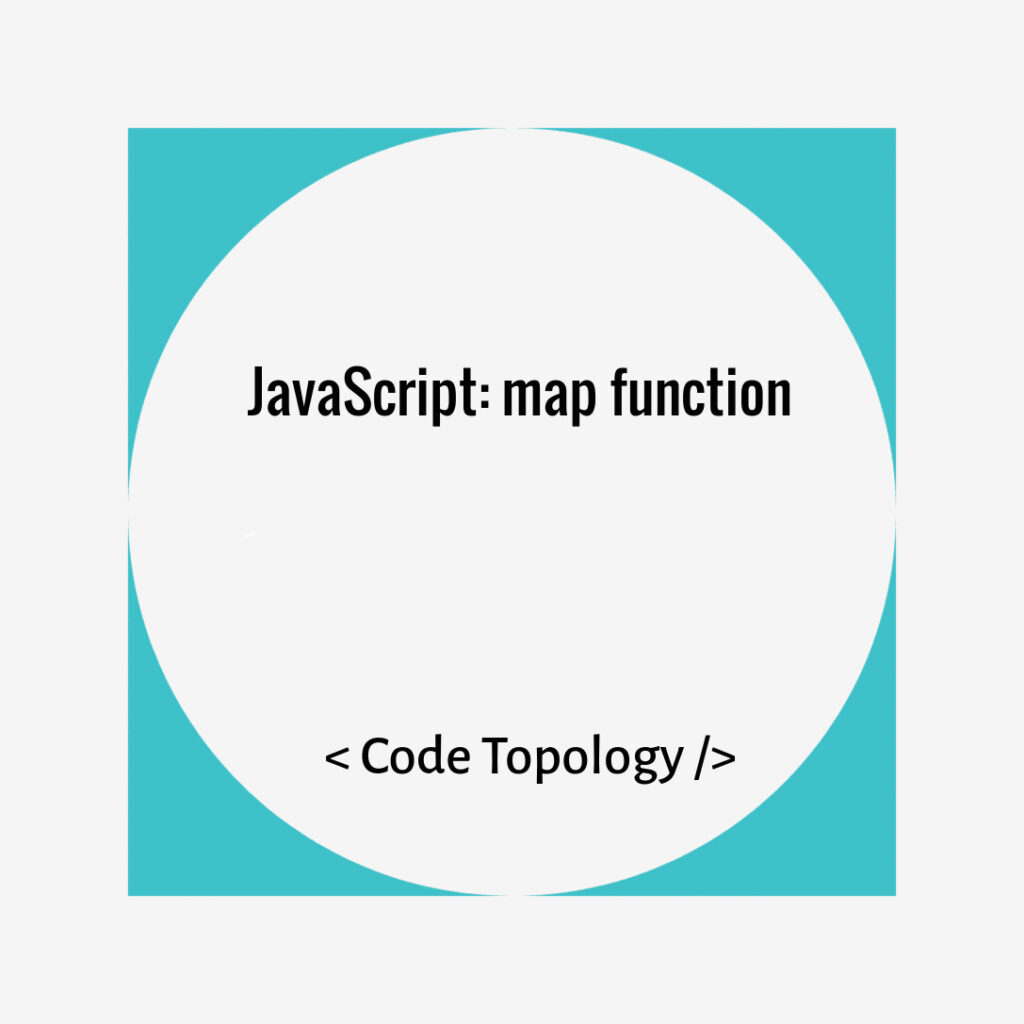
Sometimes you need to iterate through array and perform some operation and push result in new array. There are multiple ways to do it by using existing JavaScript loops but ES5 provides much cleaner and efficient way to achieve: JavaScript map function
Introduction to map function
For instance, let’s say we have students array with id and name as a field. We need to insert all ids in a new array. So you can achieve this by using traditional for loop, we can achieve this as follow,
// Students array
var students = [
{ id: 1, name: 'ABC' },
{ id: 2, name: 'BCD' },
{ id: 3, name: 'CDE' },
];
let ids = [];
for (let i = 0; i < students.length; i++) {
ids.push(students[i].id);
}
console.log(ids);
Here, output will be
[1,2,3]
As you can see, to achieve this we required to write quite amount of code and we have to keep track of current index also in our code, here it’s i
Same results can be achieved using map function in much cleaner way.
JavaScript map() Example
See the Pen VwjLYbB by Code Topology (@codetopology) on CodePen.
Here we have printed output on the console, therefore you will see a blank result screen, However, you can press F12 to open your browser’s console to see the output.
As a result of using javascript map function, we have achieved same results in quite cleaner and efficient way.
Javascript map() Syntax
arrayObj.map(callback[,context]);
arrayObj is a original array on which map() is invoked.
The map() takes 2 named arguments, First is a callback function and the second is a context object. callback function gets triggered on every element of an array.
In addition, callback function takes 3 arguments:
function callback(currentElement,index,array){
}
- currentElement – This is a current elements of array which is passed to callback function
- index – Index of the current element
- array – complete array on which map() is applied
Out of these 3 elements, currentElement parameter is mandatory while the rest 2 parameters are optional.
However, map() does not change the original array, it creates a new array element which is generated by callback function.
There is another way to write map function using arrow function provided in ES6 as follow
See the Pen javascript map function arrow function by Code Topology (@codetopology) on CodePen.
In conclusion, JavaScript map function is a modern way to iterate through an array and operate on elements in a much cleaner and efficient way.
Thank you!!