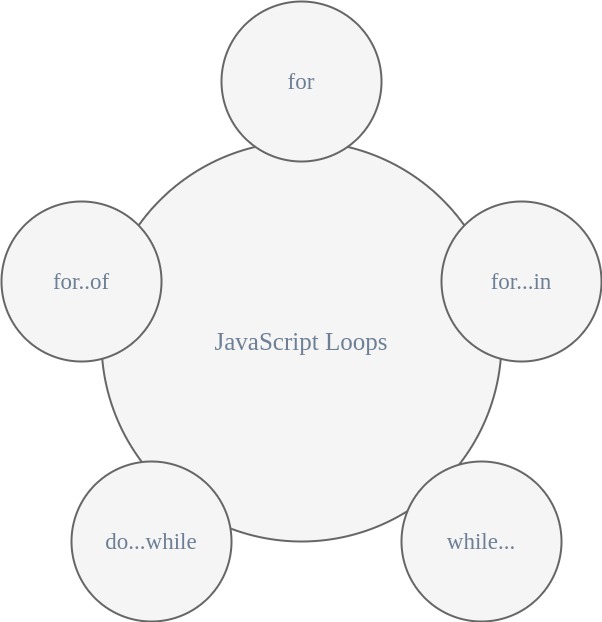
Loops in any programming language are used to execute specific set of code again and again as long as certain conditions are met. There are total 5 JavaScript loops out of which 5th loop is added in ES6 standard.
Lets take up each JavaScript loop with example.
JavaScript for loop
JavaScript for loop is a control flow statement which allows us to repeatedly execute certain code block as long as conditions are true. Whenever a number of desired executions/loops remain known, you will use this loop.
Flowchart of for loop is:
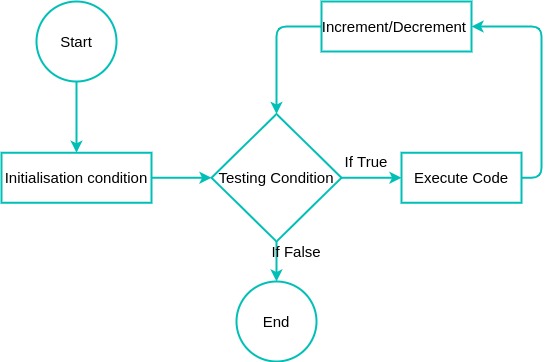
Syntax of for loop is
for(initialisation condition;testing condition ; increment/decrement)
{
//execute code
}
JavaScript for loop consist of 3 parts:
Initialisation condition
Here we initialize a variable with starting value for a loop which is also termed as a loop counter. Already declared variable serves this purpose or we can declare variable here itself. Your loop with start with a value declared here.
Testing Condition
This condition will be validated each time your loop gets executed. It must return a boolean value. Testing condition defines an exit condition for a loop
Increment / Decrement
Statement written here will update loop variable/counter value after each iteration. We can either increment counter or decrement counter.
For loop Example
<script type = "text/javaScript">
// JavaScript for loop example
var loopCounter;
for (loopCounter = 0; loopCounter <= 4; loopCounter++)
{
document.write("Iteration no "+ loopCounter);
}
< /script>
Here we have initialised loop counter with 0 value. So loop will start from 0 and will increment like 0,1,2,3,4. loopCounter <=4 specifies loop should execute only till loopCounter is less than equals to 4.
Output of above example will be:
Iteration no 0
Iteration no 1
Iteration no 2
Iteration no 3
Iteration no 4
JavaScript do….while loop
do…while loops falls under a exit control loop category because test condition will be evaluated at end of the loop therefore statements written in do while loop will get executed at least once. do…while loops are mainly used where code has to be executed at least once regardless of test condition.
Flowchart of do…. while loop is:
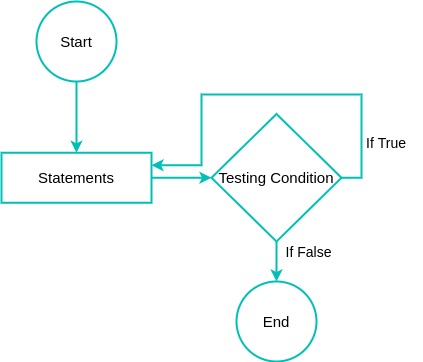
syntax of do….while loop
do {
//statements;
}while (test_condition); //Note that we have given semicolon here
Here, statements get executed first, and then test_condition is evaluated. Note that we have to manage test_condition inside do… while loop to update the counter variable. Because if this value not managed properly after each iteration, this loop will become an infinite loop.
Example of do….while
<script type = "text/javaScript">
var x = 0;
do
{
document.write("Value of x is " + x + "<br />");
x++;
} while (x < 2);
< /script>
Output of above script will be,
Value of x is 0
Value of x is 1
JavaScript while loop
while loop falls under an entry control loop category because it checks the condition at the beginning of the loop. It executes a block of code written inside parenthesis as long as the expression is true.
Flow Chart of while loop is:
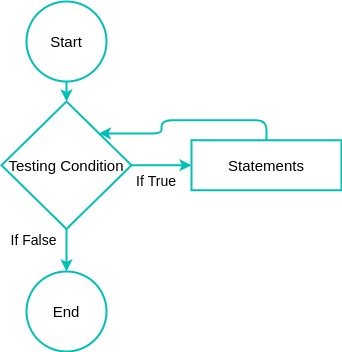
Syntax of while loop in JavaScript is
while(condition expression)
{
//statements
// increment/decrement counter
}
While loop comes with 2 constraints, the variable using in condition expression must be declared before using and increment or decrement counter must be inside the loop body.
Example of while loop
<script type = "text/javaScript">
var i =0;
while(i < 2)
{
console.log(i);
i++;
}
</script>
Output of above script will be:
0
1
JavaScript for…in loop
for…in loop is used to iterate over enumerable properties of an object. A number of iterations are equal to a number of properties in an object.
syntax of for…in loop
<script type = "text/javaScript">
for (variableName in objectName){
// statements
}
</script>
In each loop, one enumeration from objectName will be assigned to variableName.
Example of for…in loop
<script type = "text/javaScript">
var object = { name: 'abc', id: 1};
for (var v in object) {
console.log(v + " " + object[v]);
}
</script>
Output of above script will be,
name abc
id 1
JavaScript for…of loop
for…of loop is used in multiple scenarios like to iterate over array,string,map, objects etc.
Syntax of for…of loop
for(variableName of iterableName) {
// statements
}
Here comes two parts, variableName, and iterableName, in each iteration one property from iterableName, will get assigned to variableName.
Example of for…of loop
<script type = "text/javaScript">
var iterableName = ['a','b','c'];
for (value of iterableName) {
console.log(value);
}
</script>
So this is all about loops in JavaScript with an example. In the next tutorial, you will see string functions in javascript.