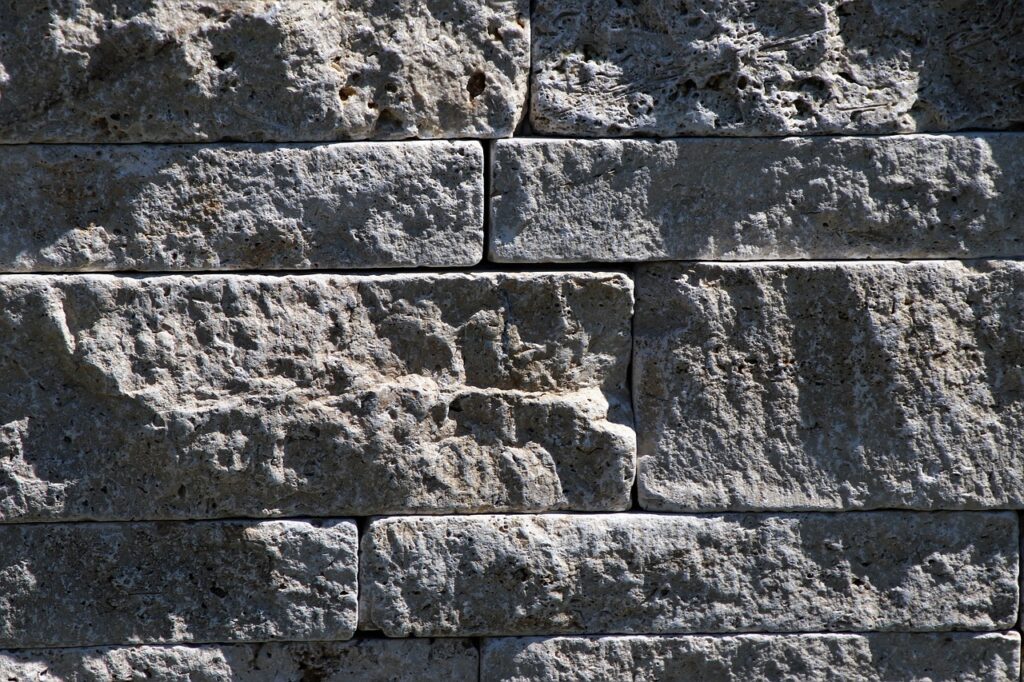
Functions, like any other language, are building blocks of javascript that allow to reuse of the code. In this article, we have already seen an inbuilt javascript function, but now we will see how we can create custom functions in javascript.
Syntax
function functionName([parameters]) {
// Function body
return value; // Optional
}
The syntax is quite simple, you need to use function keyword to declare a function. Function parameters are optional, you can specify multiple comma-separated parameters within parenthesis ( ).
Function declarations offer the advantage of hoisting, meaning they can be called before they are declared in the code.
Example
function displayName(name) {
return "Hello, " + name + "!";
}
console.log(displayName("JS")); // Output: Hello, JS!
This will print Hello, JS in console. You can use this function multiple times to display the name which demonstrates the reusability of code.
You can also assign a default value to the parameter if the caller does not pass the parameters.
function displayName(name = "JS") {
return "Hello, " + name + "!";
}
console.log(displayName()); // Output: Hello, JS!
Variable Scope
- Variables declared inside a function are local to function only, which means their scope is limited to the function they are being used.
- Functions can access and modify variables that are declared outside of it. If the same variable is declared inside a function again, then the inner variable will take precedence.
Function Expression
There is another way to define a function in javascript, and that is called function expression.
const functionName = function(parameters) {
// Function body
return value; // Optional
}
This way allows us to assign a function to a variable and pass that variable to another function as an argument. Function expressions are not hoisted, which means they must be defined before they are invoked in the code.
Now, as we know, function expression assigns a function to variable, what will happen if we print that variable? Let’s see:
const square = function(x) {
return x * x;
};
console.log(square); // Output: body of the code
The above example will print the body of the square function and will not execute it. If you wish to execute it, you may have to write
console.log(square(4)); // Output: 16
Arrow functions
There is one more way in which we can declare a function in JavaScript, and that is called the arrow function. Arrow functions are great ways to write single-line and short functions.
let functName = (arg1, arg2, ..., argN) => expression;
//or
let functName = (arg1, arg2, ..., argN) => {
return expression
};
functName is a function name, that takes arg1, arg2… argN arguments and executes expression on those argument data.
Difference between function, function expression and arrow function
- Hoisting: Function declarations are hoisted, while function expressions and arrow functions are not.
- Syntax: Function declarations have a distinct syntax with the
function
keyword, while function expressions and arrow functions offer more concise alternatives. - Flexibility: Function expressions are more flexible as they can be assigned to variables and passed around as arguments.
- Return Behavior: Arrow functions have implicit return for single expressions, while function declarations and expressions require explicit
return
statements.