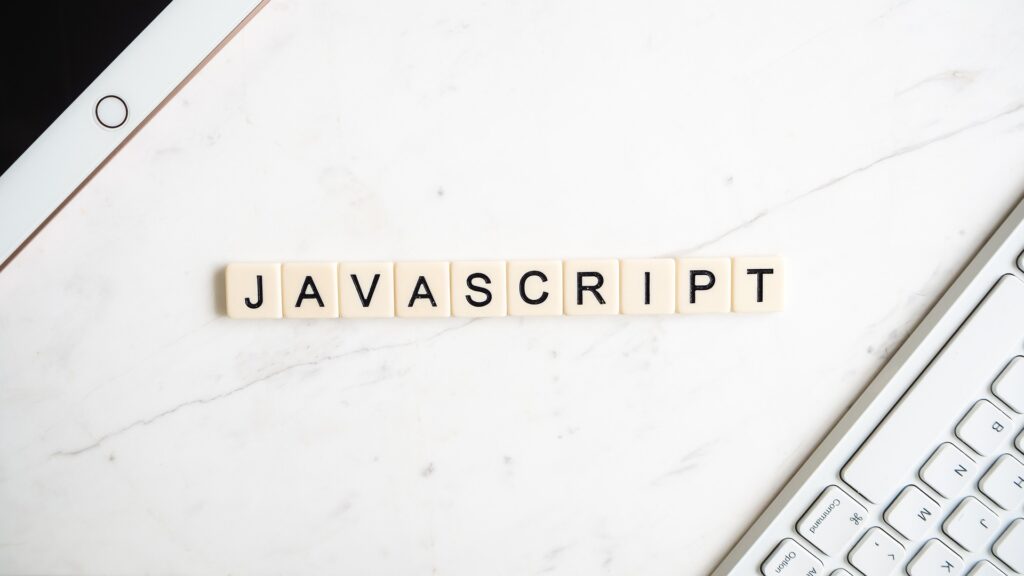
Image by Alltechbuzz_net from Pixabay
Logically, switch statement in JavaScript is nothing but a combination of if-else statements. switch statement evaluates an expression and executes a matching case block. It may or may not have default clause. Let’s see it in detail.
Syntax
switch(x) {
case 'value1':
...
[break]
case 'expression':
...
[break]
default:
...
[break]
}
value of ‘x’ will be checked against each case starting from 1st case, and if a value is matched with any case, then that block will be executed until the break statement occurs. Please note that the case clause is optional, switch statement may or may not have any case clause.
default clause is optional, it gets executed when no case is matched. switch statement in javascript will have only one default clause.
Importance of ‘break’ statement
To understand the importance of break statement, let’s see the following example first.
See the Pen Untitled by Code Topology (@codetopology) on CodePen.
The above code has 3 case clauses, which will evaluate their condition against variable ‘a’. In this case, even if ‘case 10‘: is matched and its block is executed, program flow will continue ‘case 11‘ block execution irrespective of the match.
To avoid this unexpected result, you should use ‘break‘ statement wherever needed.
Example
See the Pen Untitled by Code Topology (@codetopology) on CodePen.
As you can see, we can also use expressions in case clauses. In this case, case 5+5 will get executed.
Lexical Scope Handling
See the Pen javascript lexical scope issue by Code Topology (@codetopology) on CodePen.
The above code will throw Uncaught SyntaxError: Identifier ‘alertStatement’ has already been declared Error.
This is because variable alertStatement is already declared in case “js” clause as a const, and only one constant variable can be declared in one block scope. This means that all case clauses under switch statement share a common scope.
If there is a necessity where you need to declare const variable repeatedly in a switch statement, you can use { } brackets to define its scope as shown in the following example:
See the Pen switch lexical scope issue resolved by Code Topology (@codetopology) on CodePen.
I hope you liked the article 🙂