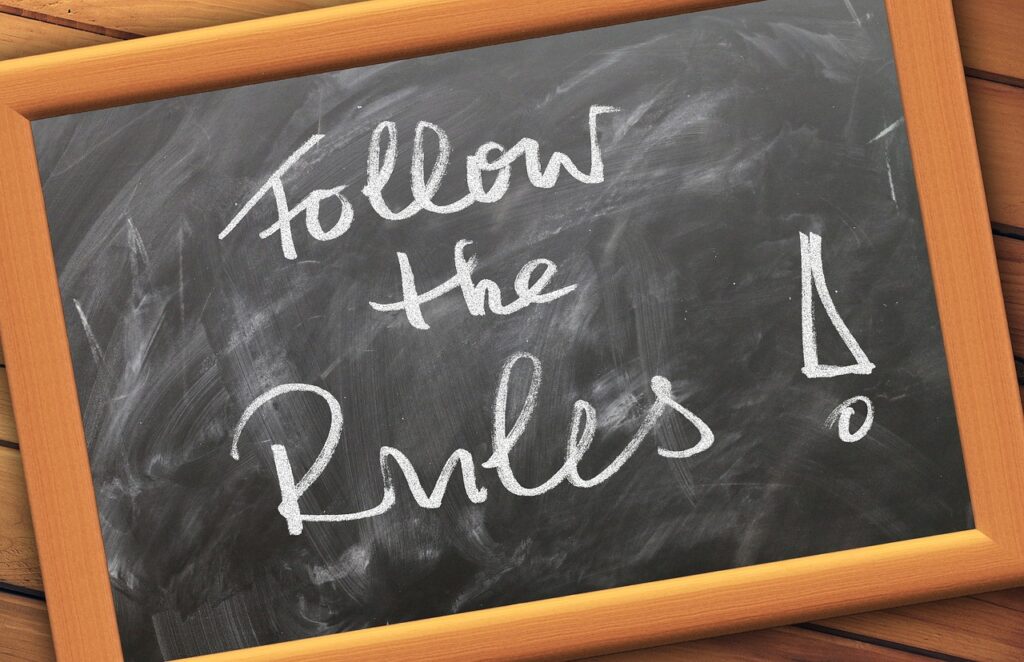
Image by Gerd Altmann from Pixabay
“use strict” mode in javascript was introduced by ECMAScript 5 which ensures that certain actions are not executed and throws additional errors that were overlooked in default javascript mode.
Why “use strict” mode?
There are multiple reasons to use strict mode in javascript:
- Strict Syntax Checking: It enforces stricter syntax rules, which helps in writing cleaner and more secure code. For instance, it disallows the use of undeclared variables.
- Preventing Silent Errors: In non-strict mode, certain actions that might lead to errors are allowed to proceed silently, potentially causing unexpected behavior. “use strict” mode helps catch and report such errors, making debugging easier.
- Compatibility: It makes sure that certain features of javascript are not used that are either deprecated or will be deprecated in future
Let’s see the working of strict mode in following article with working examples:
'use strict';
a = 'codetopology'; // throws an error
Above code will throw an error as a is not declared and used directly. Undeclared variables are not allowed in ‘use strict’.
'use strict';
car = {type: 'car', year: 1992}; // throws an error
Similar to variables, undeclared objects are not allowed in use strict mode.
'use strict';
let car = {type: 'car', year: 1992};
delete car; // throws an error
Deleting an object is not allowed in ‘use strict’ mode.
"use strict";
function car(c1, c1) { console.log('codetopology')}; // throws an error
car();
In the above code snippet, a function has two parameters with same name. This will throw an error in strict mode.
You cannot also use some reserved keywords in strict mode.
implements
interface
let
package
private
protected
public
static
yield
'use strict';
let car = {};
Object.preventExtensions(car);
// Assignment to a new property on a non-extensible object
obj.newValue = 'new value'; // throws an error
Assigning a new value to a non-extensible object is not allowed in strict mode.