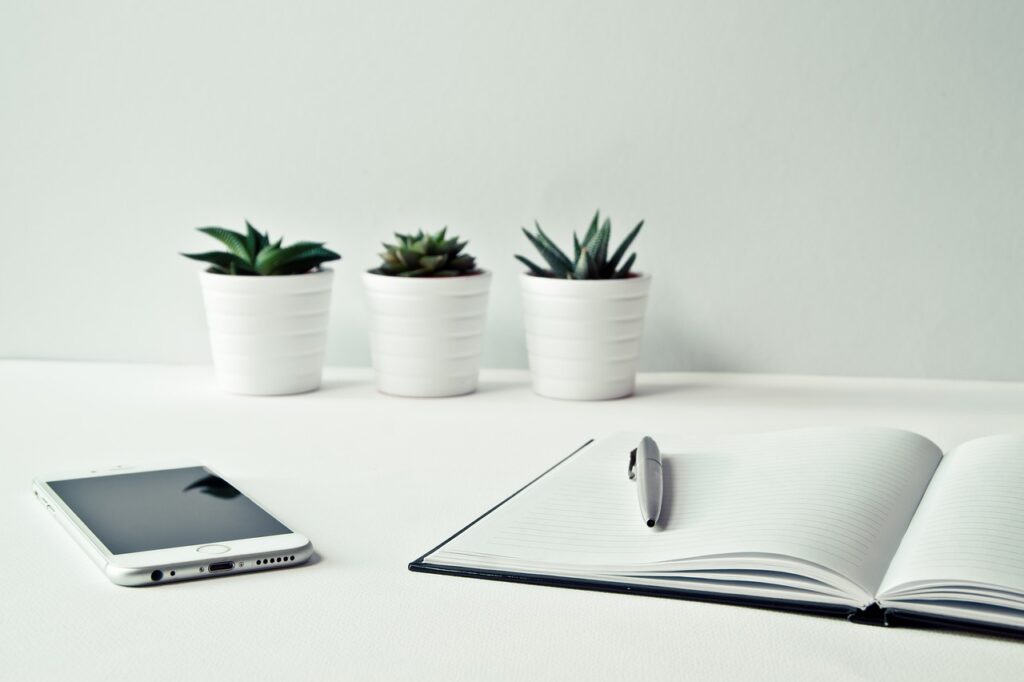
Type conversion is nothing but converting a variable from one data type to another. This process is also termed type casting. There are 2 types of type conversion in javascript:
- Implicit conversion – This happens automatically by javascript
- Explicit conversion – This is a manual type of conversion
Value | String Conversion | Number Conversion | Boolean Conversion |
---|---|---|---|
1 | “1” | 1 | true |
0 | “0” | 0 | false |
“1” | “1” | 1 | true |
“0” | "0" | 0 | true |
“five” | "five" | NaN | true |
true | “true” | 1 | true |
false | “false” | 0 | false |
null | “null” | 0 | false |
undefined | “undefined” | NaN | false |
” | “” | 0 | false |
‘ ‘ | ” “ | 0 | true |
Let’s see Javascript type conversion in detail:
Implicit Conversion to String
See the Pen Untitled by Code Topology (@codetopology) on CodePen.
You can see when number is added with string, it gets concatenated automatically. This means, number is get converted to string.
Implicit Conversion to Number
See the Pen Implicit Type Conversion Of Numbers by Code Topology (@codetopology) on CodePen.
When number is used with number with any of -, /, * these operator, it returns number.
Implicit Boolean Conversion to Number
See the Pen Implicit Conversion with Boolean by Code Topology (@codetopology) on CodePen.
JavaScript considers 0 as false
and all non-zero number as true
. And, if true
is converted to a number, the result is always 1.
Undefined with Number, Boolean or null
See the Pen Undefined with Number Boolean or null by Code Topology (@codetopology) on CodePen.
Explicit Conversion
You can transform one data type into another according to your requirements. The manual process of converting types is referred to as explicit type conversion. In JavaScript, you can perform explicit type conversions through built-in methods.
Convert to Number Manually
You can use Number() method to convert string numbers and boolean to number. null and blank string return 0. If you pass invalid string to this method, it will return NaN.
You can also use parseInt() method to convert string to number. It will return the string before the decimal point if its a string with decimal number.
Let’s see this in a working example:
See the Pen Explicit Conversion to Number by Code Topology (@codetopology) on CodePen.
Convert to String Manually
You can use String() or toString() method to convert to string data type. There is one major difference between these two methods – String() can convert null and undefined to string but toString() will return an error when null is passed.
See the Pen Explicit Conversion to String by Code Topology (@codetopology) on CodePen.
Convert to boolean manually
To convert variable to boolean data type, you need to use Boolean() method. In Javascript type conversion undefined
, null
, 0
, NaN
, ''
converts to false
where as rest all values are converted to true.
See the Pen Untitled by Code Topology (@codetopology) on CodePen.