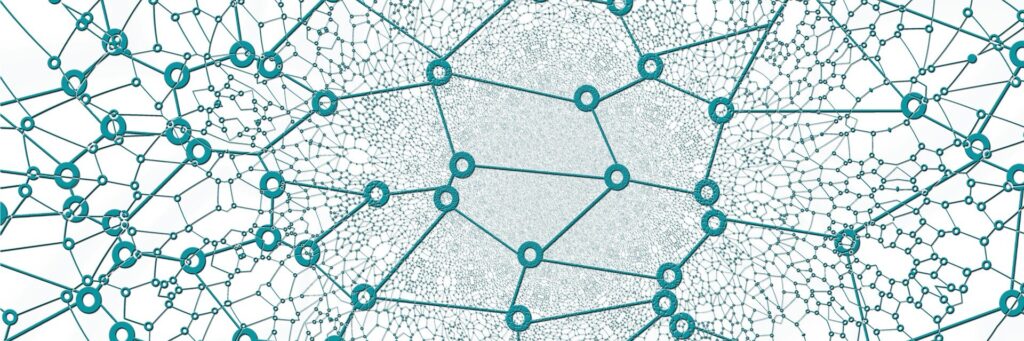
So far we have covered data types, classes in typescript. In this tutorial, we will cover typescript interfaces, what they are and how to use them with an example.
What is a TypeScript Interface ?
Interfaces are same like classes which can have methods and variables, but the difference is, there will be NO definition, only declaration. They provides basic template what class should implement, not how to implement it with detail code. Its deriving class responsibility to implement it.
How to declare Interface ?
interface my_interface_name {
}
interface keyword is used to declare an interface. Following is the example of interface.
interface User {
first_name: string,
middle_name:string,
last_name?: string // Optional Variable, not mandatory to used by derived class.
}
You can see we have declared 3 variables out of which last_name variable has ? mark after that which indicates this is not a mandatory field and can be skipped by derived class from using.
How to use it?
Lets say we have declared our interface User above. To use it, we first have to create its object as,
let user:User;
user = {first_name:"typescript", last_name:"interface"};
another example of interface with function is,
interface Vehicle{
speed(maxSpeed:number):void;
};
let tempo:Vechicle = {
speed:function (maxSpeed:Number){
//function body
}
}
Extending Interfaces
Typescript also allows extending interfaces with extends keyword, like
interface ParentInterface {
last_name:string
}
interface ChildInterface extends ParentInterface {
first_name:string
}
var fullname = <ChildInterface>{};
fullname.last_name = "Doe";
fullname.first_name = "John";
console.log("Child name is " + fullname.first_name + " " +fullname.last_name);
Output will be:
Child name is John Doe
Now we have covered class and interface in typescript, you check their fundamental difference here