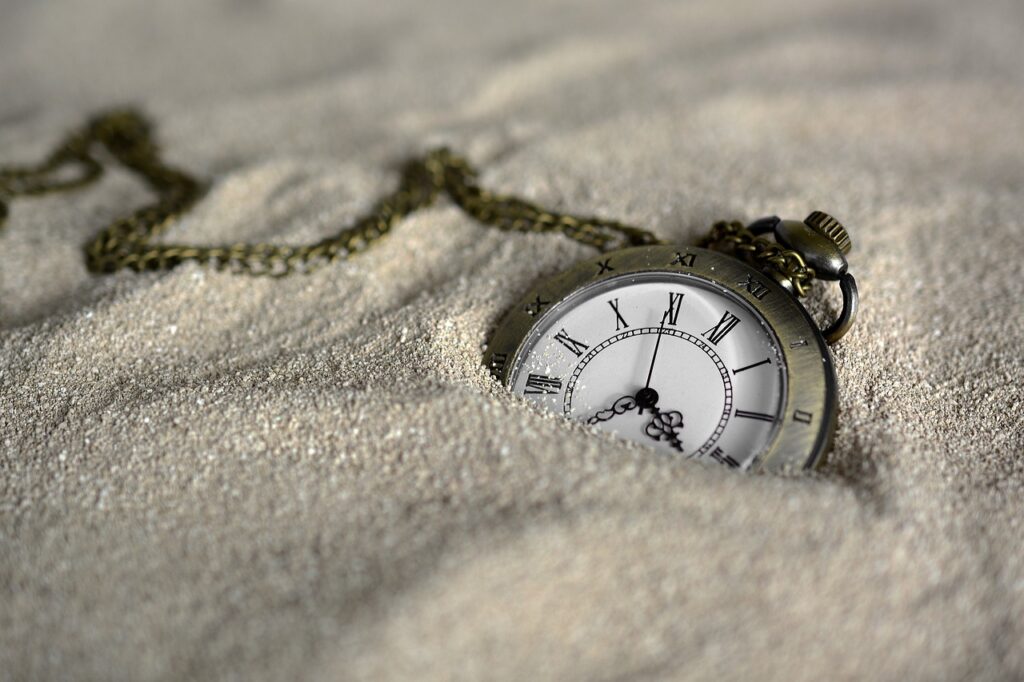
setTimeout() and setInterval() are the 2 most useful functions in JavaScript. setTimeout() in javascript is used to execute a piece of code after a specific time delay whereas setInterval() is used to repeatedly execute a piece of code or function at specified intervals.
setTimeout() Syntax
setTimeout(functionRef);
setTimeout(functionRef, delay);
Here, functionRef is a function name, that we want to execute after a specified delay. You can see in the first version of syntax above, we have not specified delay, so in this case, the function will get executed immediately.
Let’s see both examples:
setTimeout() Example
See the Pen setTimeout() in Javascript by Code Topology (@codetopology) on CodePen.
In the example, you can see we have not set a delay in
setTimeout(greetMe);
So, greetMe() function will get executed immediately upon script execution.
setTimeout() with anonymous function
You can also use setTimeout with an anonymous function. An anonymous function is passed as the argument to setTimeout.
// Using setTimeout with an anonymous function
setTimeout(function() {
console.log("This message is shown after 3 seconds");
}, 3000);
// Output: "This message is shown after 3 seconds" after a 3-second delay
setTimeout() function returns a unique identifier, which can be used to cancel the timeout event before occurring. Let’s see that:
let timeoutId = setTimeout(function() {
console.log("This will not be executed");
}, 5000);
// Cancel the scheduled timeout
clearTimeout(timeoutId);
In the above code, an anonymous function is set to execute in 5 seconds, but clearTimeout() function will cancel the scheduled execution, and console.log() will never get executed.
setInterval() Syntax
setInterval(funcRef); // variation 1
setInterval(funcRef, interval); // variation 2
You can see, syntax of setInterval and setTimeout are similar. Here, in the first variation, we have not specified interval, so the function execution will start immediately. In the second variation, the function will be executed at a specified interval.
setInterval() Example
See the Pen setInterval() in JavaScript by Code Topology (@codetopology) on CodePen.
Similarly to setTimeout, you can also use setInterval with an anonymous function:
setInterval() in anonymous function
// Using setInterval with an anonymous function
setInterval(function() {
console.log("This message is printed every 3 seconds");
}, 3000);
In this example, an anonymous function is passed as the argument to setInterval, which prints a message every 3 seconds.
Like setTimeout, the setInterval function also returns a unique identifier for the scheduled interval. This identifier can be used to cancel the repeating execution using the clearInterval function.
let intervalId = setInterval(function() {
console.log("This message is printed every 5 seconds");
}, 5000);
// Cancel the scheduled interval
clearInterval(intervalId);
In the above example, the clearInterval function is used to cancel the repeating execution of the scheduled interval. As a result, the code inside the anonymous function will no longer be executed repeatedly.
Best Practices : setTimeout() and setInterval()
To follow the best practices of using setTimeout setInterval in JavaScript, consider the following guidelines:
- Use named functions as callbacks: Instead of using anonymous functions as callbacks, define named functions for better readability, reusability, and easier debugging.
- Handle errors gracefully: Make sure to handle any potential errors that may occur within the callback functions to prevent unexpected behavior in your code.
- Be aware of the event loop’s behavior: Understand how the event loop works in JavaScript to avoid unexpected delays or blocking of code execution.
- Use setImmediate for immediate execution: If you need to execute a function in the next event loop cycle, consider using setImmediate instead of setTimeout to ensure immediate execution.
Difference Between setTimeout() and setInterval()
- Execution: The setTimeout function executes code once after a specified delay, while the setInterval function repeatedly executes code at a specified interval until it is cleared.
- Timing: setTimeout allows you to specify a delay in milliseconds before the code is executed, whereas setInterval allows you to specify an interval in milliseconds between each execution of the code.
- Control: SetTimeout provides more control over the execution of code as it allows you to execute the code after a specific delay, without repetition. On the other hand, setInterval automatically repeats the execution of code at the specified interval until it is explicitly cleared.
- ID return: setTimeout returns a unique identifier that can be used to refer to the scheduled code execution. This identifier can be used with the clearTimeout function to cancel the execution if necessary. setInterval also returns a unique identifier that can be used with the clearInterval function to stop the repeating execution.
- Use cases: setTimeout is often used for tasks such as delaying the execution of code, running animations, or implementing timeouts in asynchronous operations. setInterval, on the other hand, is commonly used for tasks that require repeated execution, such as updating the UI, polling for updates, or running periodic tasks.