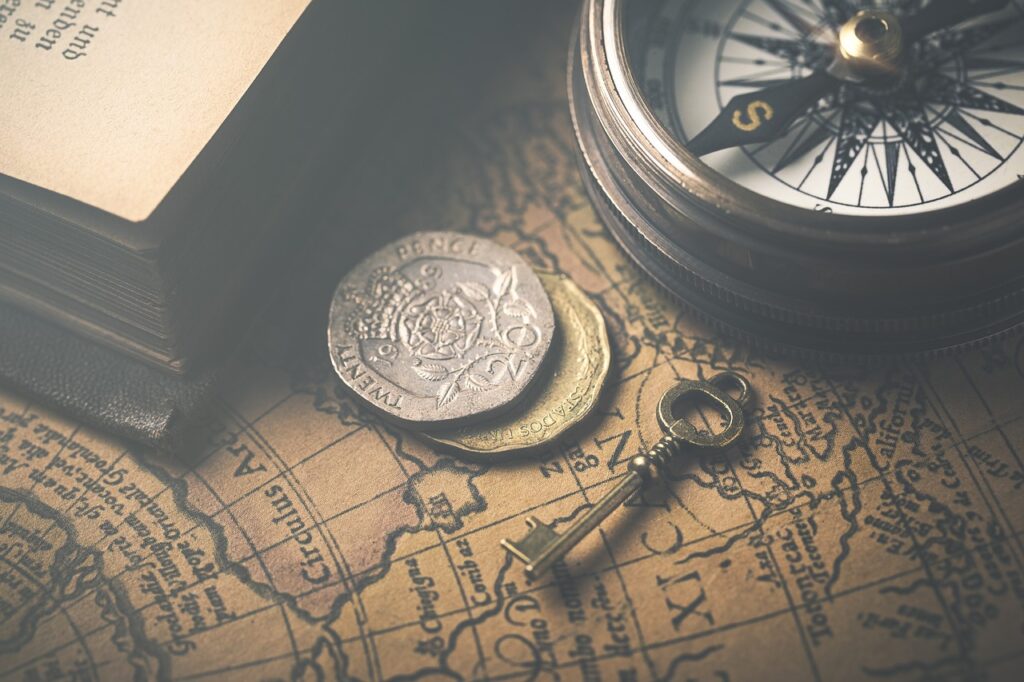
Objects in JavaScript are the most important data types that allow developers to store data,its associated functions and to organize data in key-value pairs. It allows developers to create real-world entities and more complex data structures. There are 4 ways in which we can create objects in JavaScript.
By Using Object Literals
Creating objects in JavaScript using object literals is the most common method. An object literal is defined using curly brackets {} and can contain properties and methods. Let’s see its syntax and example:
let ObjName = {key1:value1,key2:value2,"key 3":"value 3"....keyN:valueN};
As you can see in the above syntax, we can use key names with spaces by adding double quotes to that key.
Example
let person = {
name: "John",
age: 25,
occupation: "Developer",
sayHello: function() {
console.log("Hello, my name is " + this.name);
}
};
As you can see, we can also use functions inside the objects to perform the operations on the properties of that object. In the above example, we have created an object called “person” with properties like name, age, and occupation. It also has a method called “sayHello” which prints a greeting message along with the person’s name.
By using Object() Constructor
This method is less commonly used but can be useful in some situations.
Example
let car = new Object();
car.make = "Toyota car.make = "Toyota";
car.model = "Camry";
car.color = "Silver";
car.year = 2021;
In the above example, we have used the Object constructor function to create an object called “car”. We then added properties to the car object such as make, model, color, and year. This method allows us to add properties one by one after creating the object.
By Using Object.create() Method
This method allows you to create a new object using an existing object as a prototype.
Example
let person = {
name: "John",
age: 25,
occupation: "Developer"
};
let employee = Object.create(person);
employee.employeeId = 12345;
employee.salary = 5000;
In the above example, we first define an object called a “person” with properties like name, age, and occupation. Then we create a new object called “employee” using the person object as its prototype. We can then add additional properties to the employee object specific to an employee, such as employeeId and salary.
By Using ES6 Classes
ES6 supports class creation in javascript like other object-oriented programming languages. So classes can be used to create objects.
// Define a class using ES6 syntax
class Book {
constructor(title, author) {
this.title = title;
this.author = author;
}
}
// Create an instance of the Book class
const myBook = new Book("JavaScript", "John Doe");
// Display the book title and author
document.getElementById("output").innerHTML =
"Book title is : " + myBook.title + "
" +
"Book author is : " + myBook.author + "
";
Accessing Object Properties in JavaScript
We have already seen how to access object properties in javascript while learning how to create objects in javascript, but let’s see it in detail:
Dot Notation
You can access object properties using dot notation by specifying the object name followed by a dot and then the property name. For example, to access the ‘name’ property of the ‘person’ object, you can use:
console.log(person.name);
Bracket Notation
You can also access object properties using bracket notation by specifying the object name followed by square brackets containing the property name as a string. For example, to access the ‘age’ property of the ‘person’ object, you can use:
console.log(person['age']);
Both dot notation and bracket notation can be used to access properties of an object in JavaScript.
Best Practices For JavaScript Objects
- Use object literals for simple objects: When creating simple objects with a fixed set of properties, use object literals for a clean and concise syntax.
- Use object constructors for complex objects: For more complex objects or when you need to create multiple instances of an object with the same properties and methods, use object constructors.
- Use ES6 classes for object-oriented programming: ES6 introduced class syntax for creating objects in a more object-oriented way. Use classes when you need to create objects with inheritance and encapsulation.
- Use meaningful property names: Use descriptive property names that clearly indicate the purpose of each property in the object.
- Avoid mutating objects directly: Instead of directly mutating object properties, consider using methods to update object state. This can help prevent unexpected side effects.
- Use object destructuring: When working with objects, consider using object destructuring to extract values from objects and assign them to variables. This can make your code more readable and concise.
- Avoid adding properties directly to Object.prototype: Modifying the Object.prototype can have unintended consequences and lead to unexpected behavior in your code. Try to avoid adding properties directly to Object.prototype.