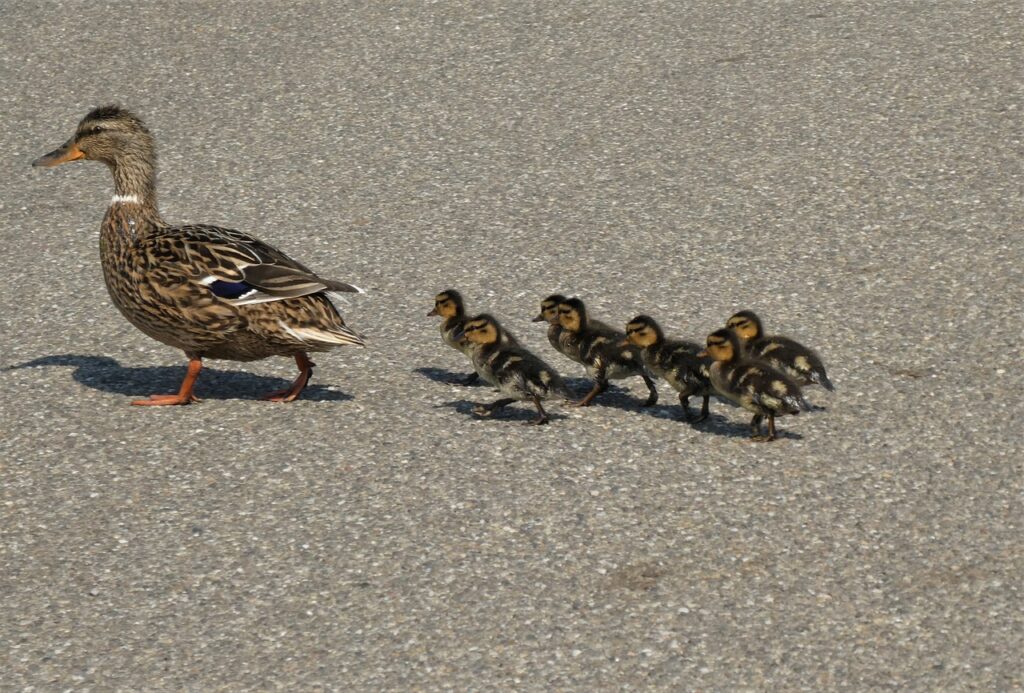
Image by Elsemargriet from Pixabay
JavaScript comments are used to add an explanatory notes in the code, to explain what code does and to disable a section or part of the code. Comments, in any language, plays an important role to make code readable and maintainable.
There are 2 types of comments in javascript.
Single Line Comment
Single-line comments start with two forward slashes //. Single line comments are ignored by the interpreter. These type of comments are used to add single line explanatory notes to the code.
Example
// This is a single-line comment
var message = "Hello, World!"; // variable initialized.
Multi-line Comment
Multi-line comments in JavaScript start with a forward slash followed by an asterisk (/) and end with an asterisk followed by a forward slash (/). Generally,multi-line comments span across a multiple lines and is used to disable a block of code. It also used to add detailed notes in the code.
Example
/*
This is a multi-line comment.
It can span across multiple lines.
These comments are often used to provide detailed explanations or to temporarily disable blocks of code.
*/
var jsTutorial = "codetopology";
If you use one multiline comment inside other multiline comment, it will cause syntax error.
In addition to these 2 types, there is one more comment type – JSDoc
JSDoc Comments
JSDoc comments are used to generate documentation from the code and are often used with tools like JSDoc or VS Code extensions. JSDoc comments start with a forward slash followed by two asterisks (/**).
/**
Calculates the sum of two numbers.
@param {number} num1 - The first number.
@param {number} num2 - The second number.
@returns {number} The sum of the two numbers.
*/
function sum(num1, num2) {
return num1 + num2;
}
JavaScript Commenting Best Practices
- Be Clear and Concise: Comments should provide clear explanations and be concise.
- Comment Before Code: It is generally recommended to write comments before the code they are explaining, as this makes the code easier to read and understand.
- Use Descriptive Comments: Use comments to explain the purpose and functionality of your code. This can help other developers (including your future self) understand your code more easily.
- Avoid Redundant Comments: Don’t repeat what the code already expresses. Comments should add value and provide additional information, not simply restate the obvious.
- Comment Complex Logic: If you have complex or intricate logic in your code, it is helpful to provide comments to explain how it works. This can make it easier to debug or modify the code later.
- Remove Unused or Outdated Comments: Regularly review your code and remove any comments that are no longer relevant. Outdated comments can be misleading and may cause confusion.
- Avoid Over-commenting: While comments are valuable, avoid excessive commenting. Code should be readable and self-explanatory as much as possible. Only add comments where they are truly necessary.
- Consider Future Developers: Keep in mind that your code might be maintained or modified by other developers in the future. Write comments that facilitate their understanding of the codebase.
- Document Function Inputs and Outputs: If you are writing a function, consider adding comments to describe the expected input parameters and return values. This helps other developers understand how to use your function correctly.
- Use proper grammar and spelling: To ensure the readability and professionalism of your code, make sure your comments have proper grammar, correct spellings, and consistent formatting.