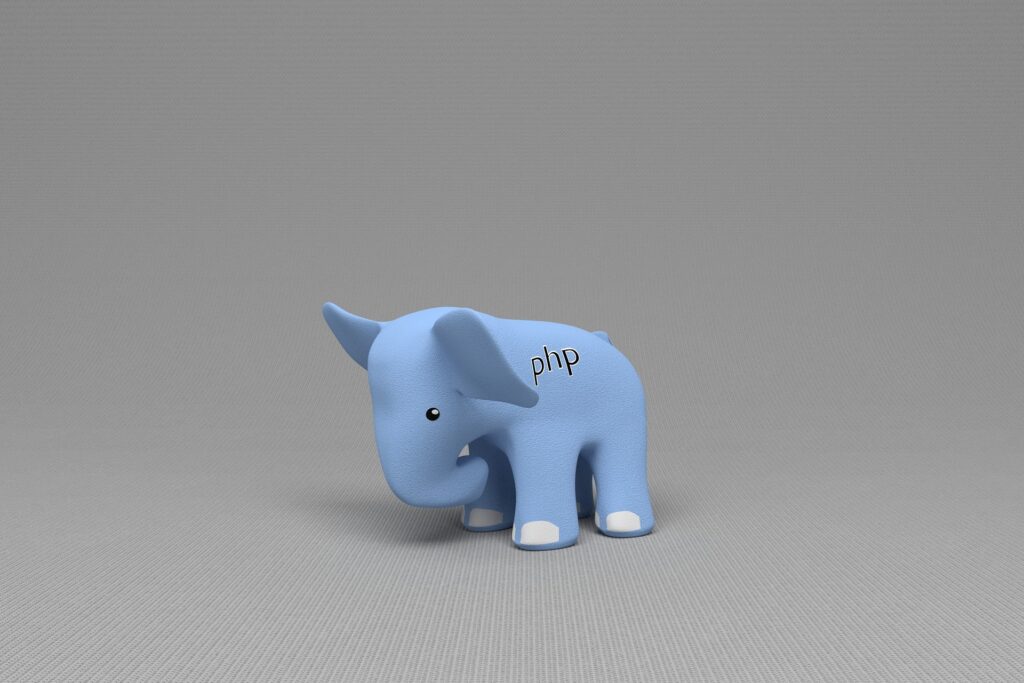
A PHP session (or any session!) is a mechanism of storing multiple values across the application pages. It’s a kind of temporary storage that gets removed as soon as session expiry time occurs.
How to start PHP session?
To start a PHP session, you must use session_start()
function on the very first line of your script.
It will enable $_SESSION global variable, which can be used to set and get session values.
How to store value in session?
To store a value in PHP session, you can use following syntax:
$_SESSION['name'] = 'MNOP';
How to get value from session?
To retrieve specific variable value from session, you can use following syntax:
$name = $_SESSION['name'];
Note that, we can also print all session values in one go using,
print_r($_SESSION);
It will print all values in array like structure.
How to modify session value?
To modify session value, use below syntax
$_SESSION['name'] = 'MNOP_updated';
Deleting specific session value
To delete a specific session value, use following syntax:
unset($_SESSION['name']);
There might be a scenario where you want to delete all session values.
To accomplish this, you can use session_destroy()
method.
Now lets take a look at example which demonstrates session get and set operations in PHP:
<?php
// Start the session
session_start();
?>
<!DOCTYPE html>
<html>
<body>
<?php
// Set session variables
$_SESSION["name"] = "Code Topology";
echo "Session variables are set.<br/>";
?>
</body>
</html>
Create one file called storeValues.php and paste above code into it. After running script, it should display “Session variables are set.”
Create another file called getSession.php which will access $_SESSION[‘name’] variable and will simply display it.
<?php
session_start();
echo $_SESSION['name'];
?>
After running above script, it should display “Code Topology” in the browser.
If you observed, we have used session_start() function on the first line in both the scripts. It is necessary to start the session access for specific script.
Hope you enjoyed the Article! 🙂