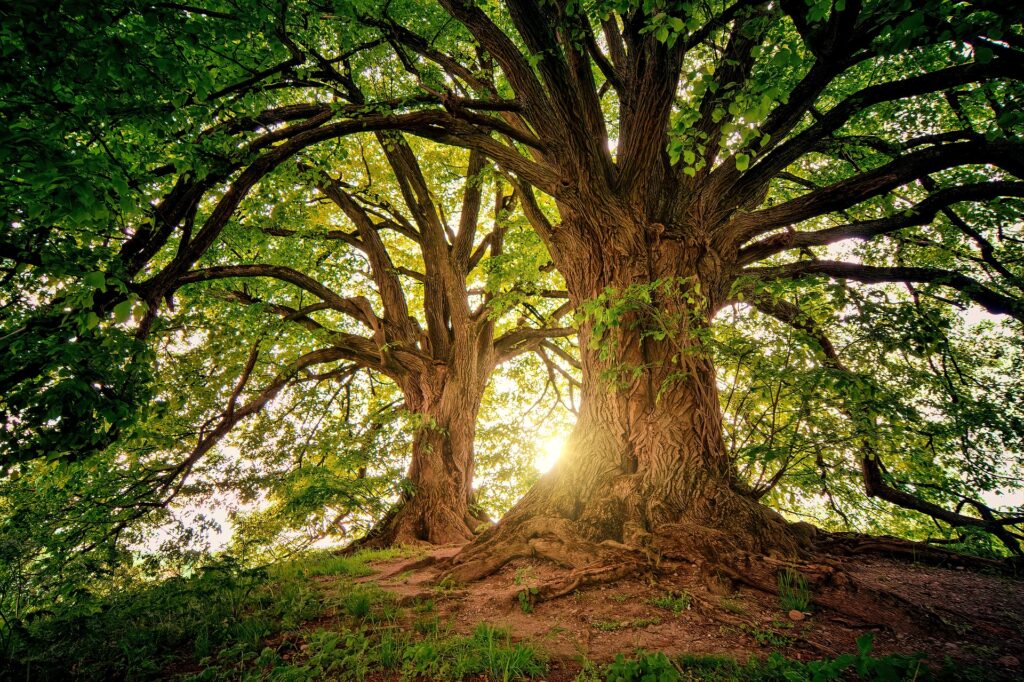
Nullish coalescing operator is a recent addition in javascript that provides an easy way to deal with null and undefined values in javascript. This is a logical operator that returns a right-hand side operand when left-hand side operand is null or undefined.
Syntax
The syntax of a null coalescing operator is written as ‘??‘.
leftExpression ?? rightExpression
Expressions can be a single variable or a complex expression or a combination of && and || operators.
For example,
The result of a ?? b
is:
- if
a
is defined, thena
, - if
a
isn’t defined, thenb
.
Example
See the Pen null operator by Code Topology (@codetopology) on CodePen.
In the first example, you can see user variable is not defined, so the ?? operator will return right hand side operand that is “Anonymous”. In this second example, as variable is defined, it will return left hand side operand that is variable admin.
Let’s see some more examples:
See the Pen advances null operator examples by Code Topology (@codetopology) on CodePen.
In example 3, as you can see, A is null is returned because of variableA is initialized as null. In example 4, even if variableB does not have any value, ?? operator will return “” as “” is not null or undefined, its just a simple empty string. In example 5, ?? returns boolean false, because false is not undefined or null, it’s a boolean falsy value.
Use cases
Determining Default Values
When working with variables that may be null or undefined, the nullish coalescing operator can be used to set a default value. For example:
const name = null;
constName = "John";
const displayName = name ?? defaultName;
console.log(displayName); // Output: John
In this example, if the name
variable is null or undefined, the defaultName
value of “John” is assigned to the displayName
.
Handling Optional Function Parameters
The nullish coalescing operator can simplify the handling of optional function parameters. Instead of using conditional statements or the ‘||’ operator to check for undefined values, we can leverage the ‘??’ operator to set default values.
function greet(name) {
name = name ?? "Friend";
console.log(Hello, ${name}!);
}
greet(); // Output: Hello, Friend!
greet("Alice"); // Output: Hello, Alice!
In this example, if the name
parameter is null or undefined, it is replaced with the default value of “Friend”.
Avoiding Repetitive Default Values
The nullish coalescing operator can help avoid repetitive default values in code. Instead of assigning the same default value multiple times, we can use the ‘??’ operator to set it once.
const defaultOptions = {
theme: "light",
fontSize: 14,
backgroundColor: "white",
};
const userOptions = {
theme: "dark",
};
const options = {
theme: userOptions.theme ?? defaultOptions.theme,
fontSize: userOptions.fontSize ?? defaultOptions.fontSize,
backgroundColor: userOptions.backgroundColor ?? defaultOptions.backgroundColor,
};
console.log(options); // Output: {theme: "dark", fontSize: 14, backgroundColor: "white"}
In this example, the ‘options’ object is created by assigning values from ‘userOptions’ object. If a value is not available, the nullish coalescing operator assigns the respective value from ‘defaultOptions’ object. This way, we avoid repeating the same default values multiple times.
Difference Between || and ?? operator
When using the || operator, 0 is considered a falsy value and will cause the operator to return the default value. However, when using the ?? operator, 0 is a defined value and will not trigger the default value to be returned.
Additionally, the precedence of the ?? operator is the same as ||, meaning it is evaluated before the assignment operator = and the ternary operator ?. This is important to keep in mind when using the operators in complex expressions to avoid unexpected results.
I hope you liked this article 🙂