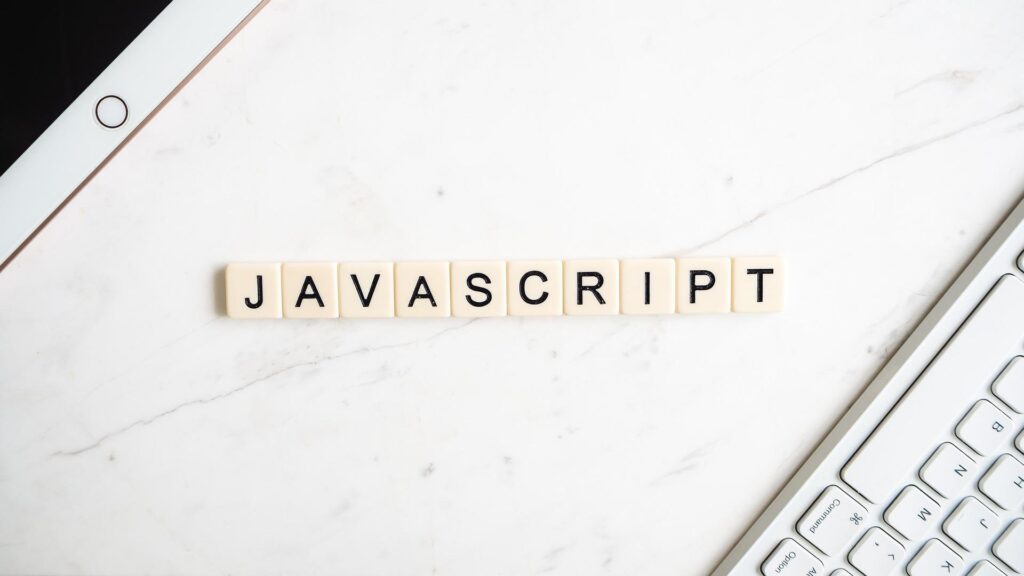
JavaScript template literals allow you to operate strings more efficiently and dynamically. Using template literals, you can implement string interpolation with embedded expression, perform operations within a string using tagged templates, and split strings into multiple lines very easily.
Template literals are enclosed by backtick ( ` ) characters instead of the single quotes or double-quotes. They are also called as template strings as they are mostly used in string interpolations. String interpolation is nothing but replacing variables and expressions with their values, which is done by the JavaScript engine.
Let’s dive into the most common usage of template literals and how they allow developers to easily deal with string operations.
String Interpolation
Let’s assume you need to combine expression output with string and display it in the console. In a traditional way, you would do something like this:
let a = 5;
let b = 5;
console.log('Five Square is ' + (a * b) + ' and\nnot ' + (a + b));
Output:
Five Square is 25 and
not 10
Imagine you have more complex expressions than (a * b ) or ( a + b ). It will become challenging to maintain such code and debug it in case you got any errors.
To avoid such concatenation using + operator with expressions and avoid overall readability issues, you can use placeholders ${expression} which are expressions delimited by $ sign and curly braces.
let a = 5;
let b = 5;
console.log(`Five Square is ${a * b} and
not ${a + b}`);
You can see we have not used any + operator and the complete string is enclosed in the simple ` character. How simple is that! No Newline character, No + operator 🙂
You just need to make sure to maintain proper indentation and white spaces. Here, ${a+b} will get replaced by 25 upon execution. We can also do the nesting of the template literals.
In the template literals, string and expression get passed to a default function if you have not provided one. If you have provided a function name explicitly, then it’s treated as tagged templates. Let’s see what is that!
Tagged Templates
Tagged templates allow you to perform an operation on template strings with function. It’s a more advanced form of the template literal. The tagged function name could be any javascript-supported valid function name.
let num = 2;
function checkNum(strings, numExp) {
let str0 = strings[0]; // "is"
let numStr;
if (numExp % 2){
numStr = 'odd number';
} else {
numStr = 'even number';
}
return `${numExp} ${str0} ${numStr}`;
}
let output = checkNum`is${num}`;
console.log(output);
// 2 is even number
Output would be:
2 is even number
Note that we have not provided any parenthesis while calling the tagged function. The first argument of a tag function contains an array of string values. The rest of the arguments are related to the expressions.
Multiline Strings
Let’s say you want to display most in-demand 5 programming/scripting languages in the console 🙂
Using normal strings, you would do
console.log('According to uplers.com 5 in-demand languages are \n' +
'JavaScript \n' +
'Html/CSS \n' +
'Python \n' +
'SQL \n' +
'Java' );
You can see for each new line we have to explicitly add newline character with multiple + operators. The same can be achieved using template literals like
console.log(`According to uplers.com 5 in-demand languages are
JavaScript
Html/CSS
Python
SQL
Java`);
As you can see the difference, using template literal made code much more readable.