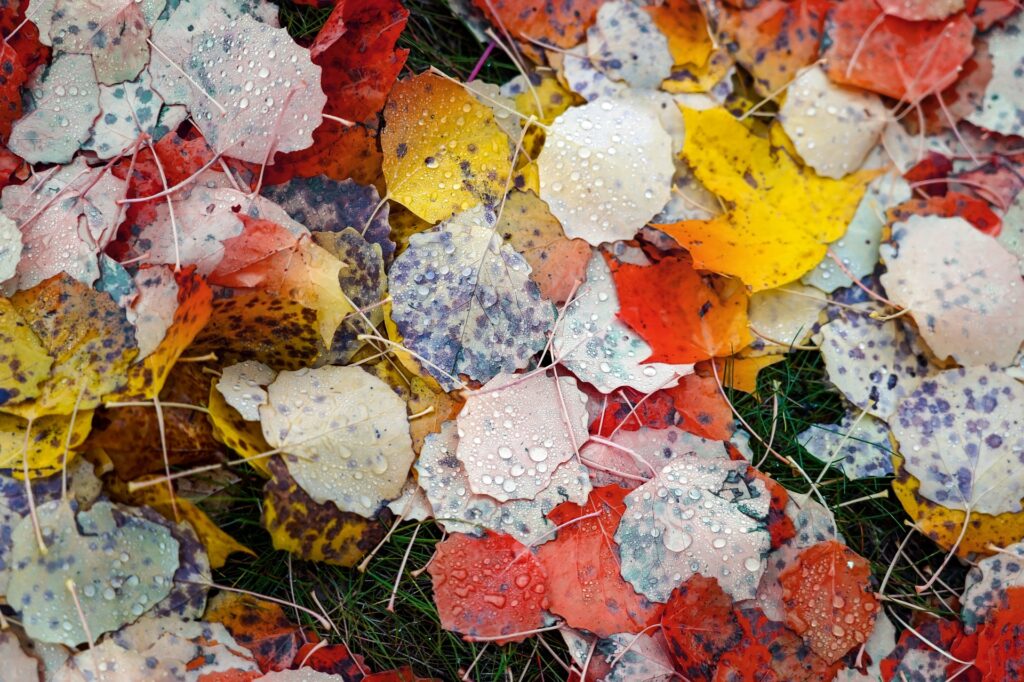
var and let both are used to declare a variable in javascript but there are few differences between these two in the below aspects.
Let’s have a look in detail:
Scope Difference
var variables scope belongs to a function if it’s defined inside a function or it belongs to global if it’s defined outside of the function.
Let’s understand these 2 scenarios:
var a; // global scoped.
function display(){
a = 5;
console.log(a);
}
In this case, the output will be:
5
As you can see, a is declared outside of the function, so it can be accessed anywhere in the script. Its scope belongs to a global.
Let’s see an example of a function scope variable:
function display(){
var a = 5;
console.log(a);
}
console.log("outside value is "+ a); //value of a will be undefined
The output will be:
5
outside value is undefined
In the above example, we have declared a variable inside a function, that is why its scope is function scope, and it shows value as undefined when accessed outside of the function.
let variables are blocked scopes. Blocks are nothing but a group of statements written inside a {} pairs. It means they can be accessed only inside a block where they are declared.
Let’s see its example:
let message = "message from global";
let limit = 1;
if (limit < 2) {
let reply = "message from local";
console.log(reply);// "message from local"
}
console.log(reply) // reply is not defined
Here we can see, the reply variable is declared inside a function block, so its scope is limited to function only.
When let variable is accessed outside of the function, it’s showing undefined.
Re-declaration
var keyword allows you to re-declare a variable whereas let keyword will not allow you to do it.
var message = "Hi";
console.log(message);
function overwriteMessage(){
var message = "Overwritten";
}
console.log(message);
Output of this will be:
Hi
Overwritten
You can see the value of the message variable got changed even if assignment is done inside of the function.
let message = "Hi";
console.log(message);
function overwriteMessage(){
let message = "Overwritten"; //will throw an error
}
console.log(message);
Accessibility through global objects
The global var variables are added to the window object of the web browser and global object of node.js.
So that they can be accessed using window or global object.
var message = "hi";
console.log(window.message); //hi
However, let variables are not accessible through global objects.
Temporal Dead Zone
var variables do not have temporal dead zone whereas let variables do have. Every variable has 2 steps in its lifecycle – Creation, and Execution.
In var variables case, when the variable is declared, storage space is assigned and value is initialized to undefined if no other value is specified.
But in the case of let, when the variable is declared, storage space is assigned but it’s not initialized, not even with the undefined value.
That why when we access a let variable without doing any value assignment, it throws a ReferenceError.
One thought